top of page
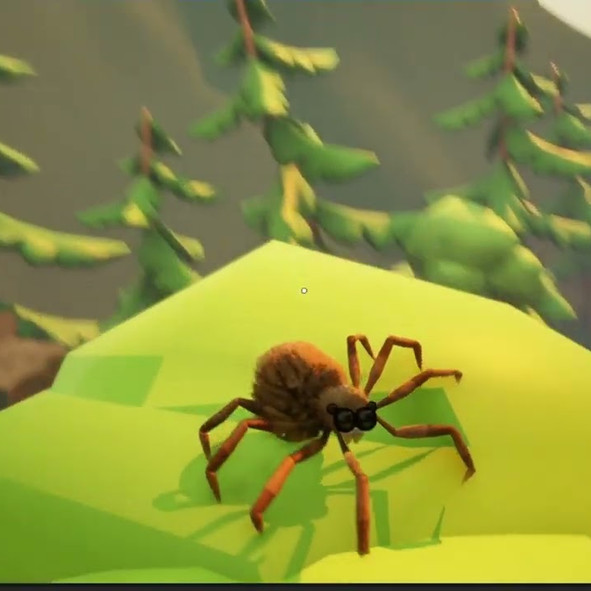
Unreal Engine 5 Game
- Developed a game prototype from scratch in Unreal Engine 5 in a small team.
- Focused on core integration, global state systems, level design, and SFX.
- Worked with procedural animation system for spider.
- Managed team through playtests, goal prioritization, and extensive documentation.
- Focused on core integration, global state systems, level design, and SFX.
- Worked with procedural animation system for spider.
- Managed team through playtests, goal prioritization, and extensive documentation.
Houdini Plug-in Tool
In this group project, we made a Houdini plug-in tool that accurately simulates how water and vegetation interact together to produce an ecosystem.
For this project, I implemented the full back-end of the system that controls the simulation, based on this SIGGRAPH paper:
https://dl.acm.org/doi/abs/10.1145/3528223.3530146
There are many potential uses for this tool, including an accurate demonstration of the impact of climate change in order to bring about an increased understanding of global environmental issues. This plug-in also allows users to visualize local ecoclimate phenomena. We expect artists and game designers to use this tool to model a natural environment. The changing environment created by this tool creates an immersive experience, so the artist can show how their environment will change as time progresses.
For this project, I implemented the full back-end of the system that controls the simulation, based on this SIGGRAPH paper:
https://dl.acm.org/doi/abs/10.1145/3528223.3530146
There are many potential uses for this tool, including an accurate demonstration of the impact of climate change in order to bring about an increased understanding of global environmental issues. This plug-in also allows users to visualize local ecoclimate phenomena. We expect artists and game designers to use this tool to model a natural environment. The changing environment created by this tool creates an immersive experience, so the artist can show how their environment will change as time progresses.
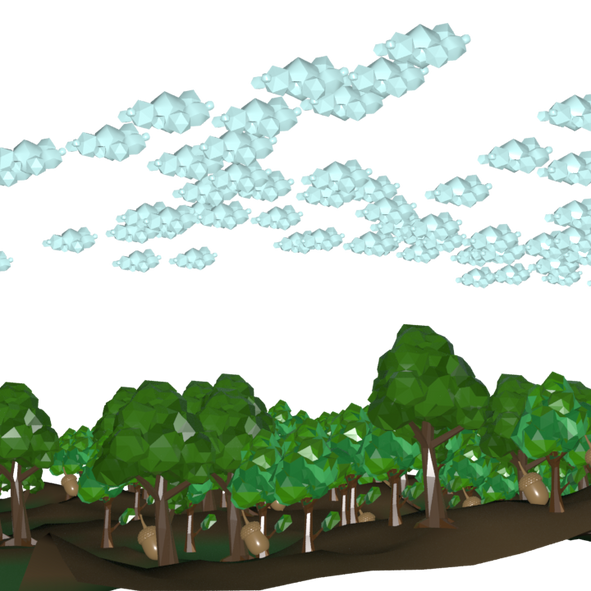
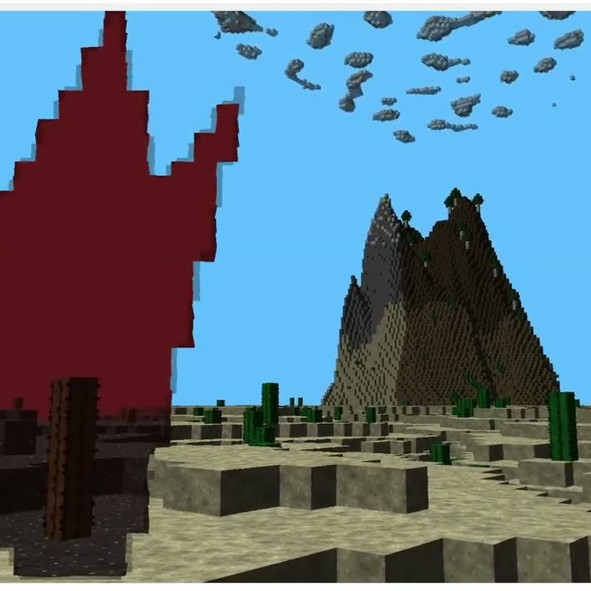
Mini-Minecraft
This was a group project in which a small team of two others and I
built a mini-version of the popular game Minecraft
from scratch in C++ and GLSL.
Our project was accepted into our professor's "Hall of Fame!"
My contributions included:
- Physics Engine
(collision, interacting with terrain, flight mode, walking mode, jumping)
- Texturing
(pulling from texture atlas, alpha blending, separating
VBOs for transparency, animating textures)
- Procedurally Generating Additional Biomes
(using noise functions like Worley, FBM, and Perlin, I determined terrain/biomes using elevation and moisture)
- Procedurally Generating Assets
(procedurally added assets to the terrain
like trees, bushes, cacti, icicles, and clouds).
Helped teammates with their features:
- Multi-threading,
- Frame buffering for post-process shaders in water and lava, and
- Chunking to increase rendering efficiency
built a mini-version of the popular game Minecraft
from scratch in C++ and GLSL.
Our project was accepted into our professor's "Hall of Fame!"
My contributions included:
- Physics Engine
(collision, interacting with terrain, flight mode, walking mode, jumping)
- Texturing
(pulling from texture atlas, alpha blending, separating
VBOs for transparency, animating textures)
- Procedurally Generating Additional Biomes
(using noise functions like Worley, FBM, and Perlin, I determined terrain/biomes using elevation and moisture)
- Procedurally Generating Assets
(procedurally added assets to the terrain
like trees, bushes, cacti, icicles, and clouds).
Helped teammates with their features:
- Multi-threading,
- Frame buffering for post-process shaders in water and lava, and
- Chunking to increase rendering efficiency
Computer Animation
3D Math Projects
Tools: Linear Algebra, C++, Building Unity Plugins, Houdini
This shows a series of projects including
- Splines with Linear Interpolation, Bernstein-Bezier curves,
Decastlejau, Hermite, Matrix curves
- Forward Kinematics
- Inverse Kinematics with Limb-based approach and CCD algorithm
- Motion Capture that I recorded and linearly blended
- Particle Simulation with euler integration and 2nd order runge kutta
Tools: Linear Algebra, C++, Building Unity Plugins, Houdini
This shows a series of projects including
- Splines with Linear Interpolation, Bernstein-Bezier curves,
Decastlejau, Hermite, Matrix curves
- Forward Kinematics
- Inverse Kinematics with Limb-based approach and CCD algorithm
- Motion Capture that I recorded and linearly blended
- Particle Simulation with euler integration and 2nd order runge kutta

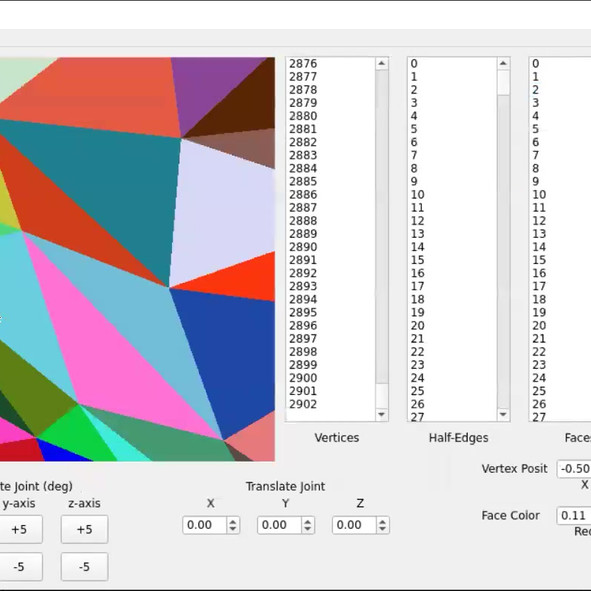
Building Mini Maya Project
In this project, I built a "mini" version of Maya
from basic fundamentals in C++.
This is a 3D object builder and manipulator. I started by implementing a half-edge
Mesh data structure, where you can manipulate face,
vertex, and edge data.
Then, you can use mesh operations, like
subdivide, which works just like Maya's Mesh smooth, to quickly
smooth the mesh.
In this project, I also implemented a skinning algorithm. The user can load mesh data, which it parses into a model, and a skeleton that will skin the mesh.
from basic fundamentals in C++.
This is a 3D object builder and manipulator. I started by implementing a half-edge
Mesh data structure, where you can manipulate face,
vertex, and edge data.
Then, you can use mesh operations, like
subdivide, which works just like Maya's Mesh smooth, to quickly
smooth the mesh.
In this project, I also implemented a skinning algorithm. The user can load mesh data, which it parses into a model, and a skeleton that will skin the mesh.
GPU Waterfall Particle Simulation
LIVE DEMO: https://kyrasclark.github.io/final-project/
In this project, I made a GPU-based particle simulation. This project is a fully physically-based particle sim with collisions all on the GPU. The particles work with transform feedback shaders, instanced rendering, and billboards, and the obstacles work with frame buffers and textures. This allows for the full system to work highly efficiently in linear time.
The user has the following controls that allow them to control the system:
- Particle Color
- Number of Particles
- Size of the Particles
- Size of the Obstacle
- Shape of the Obstacle (star or circle)
- Visibility of the Obstacles
- Camera control
- Gravity control
- Wind control (with noise option)
- Control over the generation of the particles (with FBM noise, amplitude and frequency, allowing for control over where the particles stream into the scene)
In this project, I made a GPU-based particle simulation. This project is a fully physically-based particle sim with collisions all on the GPU. The particles work with transform feedback shaders, instanced rendering, and billboards, and the obstacles work with frame buffers and textures. This allows for the full system to work highly efficiently in linear time.
The user has the following controls that allow them to control the system:
- Particle Color
- Number of Particles
- Size of the Particles
- Size of the Obstacle
- Shape of the Obstacle (star or circle)
- Visibility of the Obstacles
- Camera control
- Gravity control
- Wind control (with noise option)
- Control over the generation of the particles (with FBM noise, amplitude and frequency, allowing for control over where the particles stream into the scene)
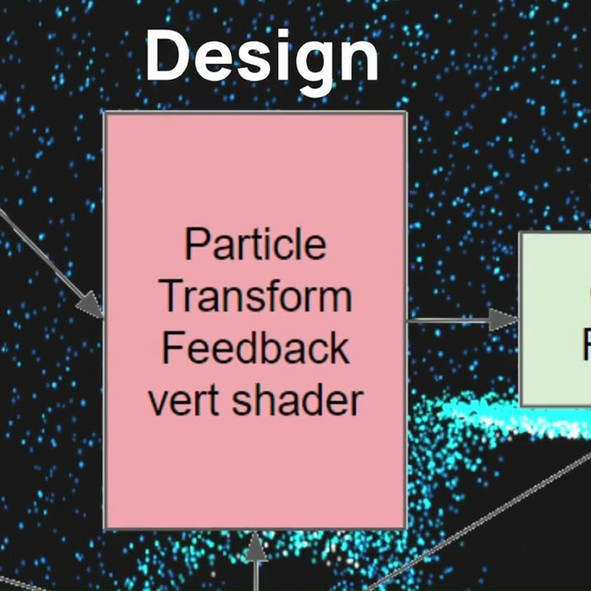
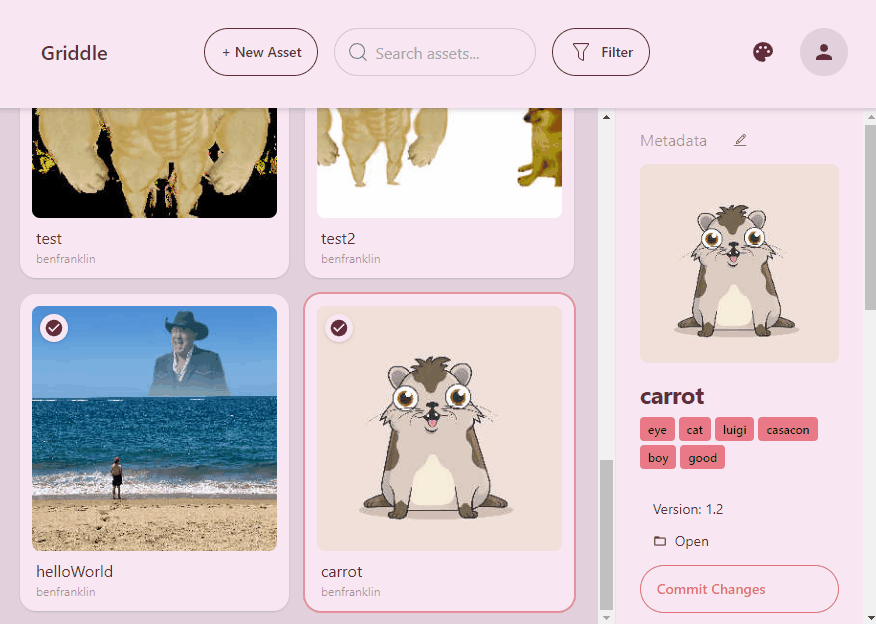
USD Full Stack Pipeline
Built a full stack USD asset pipeline, allowing users to upload, sort, edit, and download assets with version control in Python and Typescript/React.
Served as lead developer in pipeline migration between team’s 3 DB backends, rest API accessors, and front-end web applications features.
Wrote documentation for migration proposal.
Architected and implemented full stack version control tools for users to organize, edit, and share USD assets.
Served as lead developer in pipeline migration between team’s 3 DB backends, rest API accessors, and front-end web applications features.
Wrote documentation for migration proposal.
Architected and implemented full stack version control tools for users to organize, edit, and share USD assets.
Motion Capture
For this project, I worked through the whole motion capture pipeline.
I captured the mocap of myself, process all the data, and applied animation blending to blend difference motions together to create a smooth result.
I captured the mocap of myself, process all the data, and applied animation blending to blend difference motions together to create a smooth result.
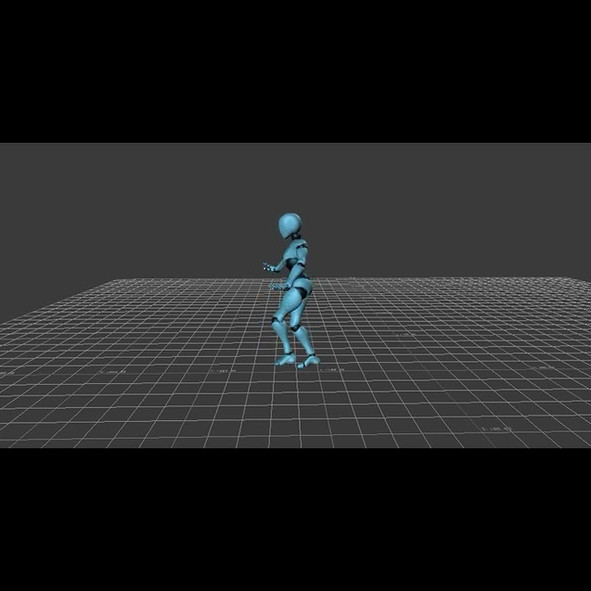

L-System Maya Plug-in
For this project, I built a Maya Plug-in to easily create the geometry for an L-System Tree.
It has a GUI and adds a button to the menu bar. Users can either directly add information to the GUI to create a static tree, or they may create a dynamic L-System Node that will update and preview the tree with the parameters from the user and will grow the tree, unrolling the L-System over time.
To further expand this project, I would like to test with more complicated geometry and L-Systems, as well as adjusting the parts of the tree manually with different parameters.
It has a GUI and adds a button to the menu bar. Users can either directly add information to the GUI to create a static tree, or they may create a dynamic L-System Node that will update and preview the tree with the parameters from the user and will grow the tree, unrolling the L-System over time.
To further expand this project, I would like to test with more complicated geometry and L-Systems, as well as adjusting the parts of the tree manually with different parameters.
Online Shopping in VR Prototype
Shopify, Inc.
HMC Clinic Project, 2021–22
Skills: A-Frame, Unity, R&D, Rapid Prototyping, Project Manager
In order to stay at the forefront of e-commerce innovation,
this project intends to explore how virtual reality (VR) technology
will transform online shopping. Our goal is primarily based
on ideation and rapid prototyping to develop a VR e-commerce
experience. We focused on exploring many ideas, but honed
in on building a single proof-of-concept prototype
showcasing how VR e-commerce could add novel interactions
to product discovery, product investigation, and merchant platforms.
HMC Clinic Project, 2021–22
Skills: A-Frame, Unity, R&D, Rapid Prototyping, Project Manager
In order to stay at the forefront of e-commerce innovation,
this project intends to explore how virtual reality (VR) technology
will transform online shopping. Our goal is primarily based
on ideation and rapid prototyping to develop a VR e-commerce
experience. We focused on exploring many ideas, but honed
in on building a single proof-of-concept prototype
showcasing how VR e-commerce could add novel interactions
to product discovery, product investigation, and merchant platforms.

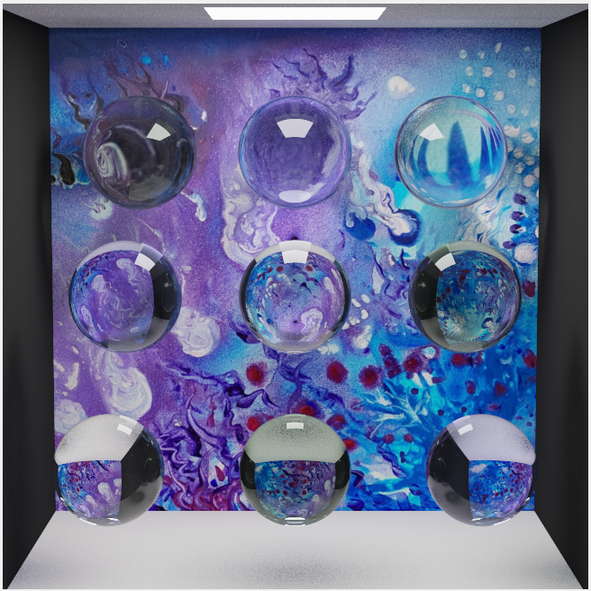
CPU Monte Carlo Pathtracer
Built real-time full lighting integrator on GPU & CPU with naive, direct, and global illumination.
Rendered diffuse, reflective, transmissive materials, and Microfacet BRDFs.
Used Russian Roulette ray termination and Multiple Importance Sampling.
Rendered diffuse, reflective, transmissive materials, and Microfacet BRDFs.
Used Russian Roulette ray termination and Multiple Importance Sampling.
GLSL Shaders with Environment Map
Implemented the PBR Unreal Engine 4 pipeline on Qt application with OpenGL based on paper “Real Shading in Unreal Engine 4”.
Used GGX/Trowbridge-Reitz methods.
Implemented specular image-based lighting on Cook Torrance microfacet material.
Developed real-time variable roughness and metallic properties.
Used GGX/Trowbridge-Reitz methods.
Implemented specular image-based lighting on Cook Torrance microfacet material.
Developed real-time variable roughness and metallic properties.
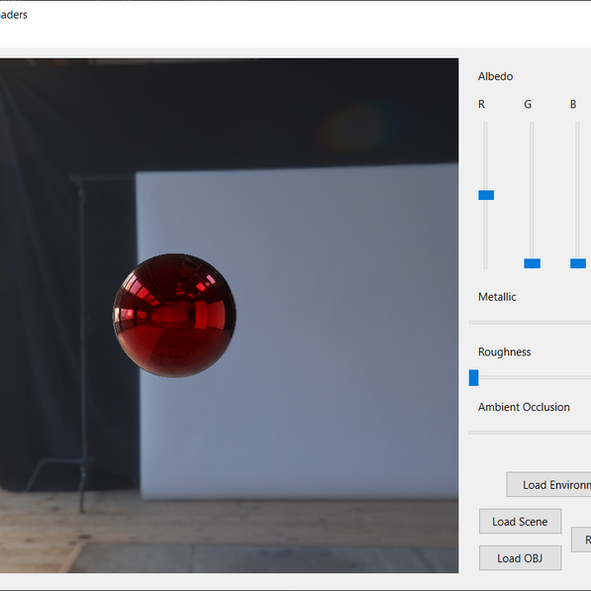
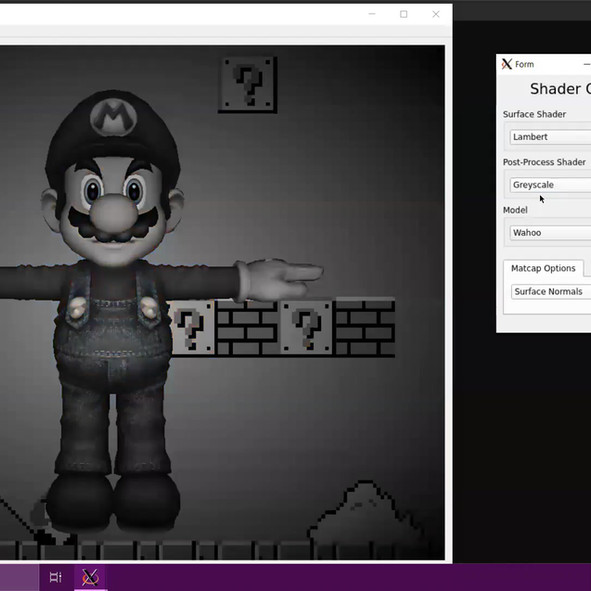
OpenGL Shaders
In this project, I built several vertex surface shaders, fragment
surface shaders, and post-process shaders in the OpenGL pipeline for
this model of Mario.
The shaders included are:
A non-uniform position and color, Lambertain shading, Blinn-Phong
shading, several matte texture , iridescent color gradient, greyscale,
vingette, Gaussian blur, Sobel filter, and a variation of a bloom and
pointillism shader.
surface shaders, and post-process shaders in the OpenGL pipeline for
this model of Mario.
The shaders included are:
A non-uniform position and color, Lambertain shading, Blinn-Phong
shading, several matte texture , iridescent color gradient, greyscale,
vingette, Gaussian blur, Sobel filter, and a variation of a bloom and
pointillism shader.
Building a Rasterizer
In this project, I built a rasterizer for drawing scenes composed of 2D
and 3D polygons. To begin, I developed a 2D triangle rasterization
algorithm. Then, I implemented a perspective projection
camera in order to project 3D polygons down into 2D space to be
rasterized. This video shows a demo of that 3D rasterizer, showing a
cube with texture, two polygons with z-buffering, and a 3D Mario
model with Lambertian shading.
and 3D polygons. To begin, I developed a 2D triangle rasterization
algorithm. Then, I implemented a perspective projection
camera in order to project 3D polygons down into 2D space to be
rasterized. This video shows a demo of that 3D rasterizer, showing a
cube with texture, two polygons with z-buffering, and a 3D Mario
model with Lambertian shading.

bottom of page